☰
Crossing clock domains 2 - Flag
A flag to another clock domain
If the signal that needs to cross the clock domains is just a pulse (i.e. it lasts just one clock cycle), we call it a "flag".
The previous design usually doesn't work (the flag might be missed, or be seen for too long, depending on the ratio of the clocks used).
We still want to use a synchronizer, but one that works for flags.
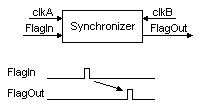
The trick is to transform the flags into level changes, which can more easily cross clock domains.
module Flag_CrossDomain(
input clkA,
input FlagIn_clkA, // this is a one-clock pulse from the clkA domain
input clkB,
output FlagOut_clkB // from which we generate a one-clock pulse in clkB domain
);
reg FlagToggle_clkA;
always @(posedge clkA) FlagToggle_clkA <= FlagToggle_clkA ^ FlagIn_clkA; // when flag is asserted, this signal toggles (clkA domain)
reg [2:0] SyncA_clkB;
always @(posedge clkB) SyncA_clkB <= {SyncA_clkB[1:0], FlagToggle_clkA}; // now we cross the clock domains
assign FlagOut_clkB = (SyncA_clkB[2] ^ SyncA_clkB[1]); // and create the clkB flag
endmodule
Now if you want the clkA domain to receive an acknowledgment (that clkB received the flag), just add a busy signal.
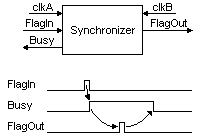
module FlagAck_CrossDomain(
input clkA,
input FlagIn_clkA,
output Busy_clkA,
input clkB,
output FlagOut_clkB
);
reg FlagToggle_clkA;
always @(posedge clkA) FlagToggle_clkA <= FlagToggle_clkA ^ (FlagIn_clkA & ~Busy_clkA);
reg [2:0] SyncA_clkB;
always @(posedge clkB) SyncA_clkB <= {SyncA_clkB[1:0], FlagToggle_clkA};
reg [1:0] SyncB_clkA;
always @(posedge clkA) SyncB_clkA <= {SyncB_clkA[0], SyncA_clkB[2]};
assign FlagOut_clkB = (SyncA_clkB[2] ^ SyncA_clkB[1]);
assign Busy_clkA = FlagToggle_clkA ^ SyncB_clkA[1];
endmodule